四、spring整合springMVC
1、配置web.xml
- 全局配置
<!--全局配置,加载spring配置文件-->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath*:applicationContext.xml</param-value>
</context-param>
- 配置监听器
<!--配置监听器,web启动就开始读取配置-->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
- 配置前端控制器
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath*:springmvc.xml</param-value>
</init-param>
</servlet>
<!--配置为rest风格-->
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
- 配置过滤器
<filter>
<filter-name>CharsetFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>CharsetFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<!--全局配置,加载spring配置文件-->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath*:applicationContext.xml</param-value>
</context-param>
<!--配置监听器,web启动就开始读取配置-->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<!--1. 配置前端控制器-->
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath*:springmvc.xml</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<!--2. 过滤编码-->
<filter>
<filter-name>CharsetFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>CharsetFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
2、设计表示(controller)层,添加@RestController注解
- 其他注解功能
注解名称 | 功能 |
---|---|
@RequestMapping | 配置 Web 请求的映射 |
@RequestParam | 接收前端传递给后端的普通类型数据 |
@RequestBody | 接收前端传递给后端的json中的数据 |
@PathVariable | 绑定占位符参数到方法参数中 |
package com.godgy.controller;
import com.godgy.pojo.Book;
import com.godgy.service.BookService;
import com.godgy.utils.PageResult;
import com.godgy.utils.Result;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
/**
* @author : Mr.Godgy
* @version : 1.0
* @date : 2022/8/13
* @description:
*/
@RestController
//要配置 Web 请求的映射
@RequestMapping("/book")
public class BookController {
private BookService bookService;
@Autowired
public void setBookService(BookService bookService) {
this.bookService = bookService;
}
@RequestMapping("/findByPage")
public PageResult<Book> findByPage(@RequestParam(defaultValue = "1") int page,
@RequestParam(defaultValue = "2") int size){
return bookService.findByPage(page,size);
}
@RequestMapping("/findById/{bid}")
public Result findById(@PathVariable Integer bid){
Book book = bookService.findById(bid);
if (book == null){
return new Result(500,"获取失败");
}
else {
return new Result(200,"成功获取",book);
}
}
@RequestMapping("/update")
public Result update(@RequestBody Book book){
System.out.println(book);
return new Result(200,"ok");
}
}
3、配置springmvc
- 配置扫描controller
<context:component-scan base-package="com.godgy">
<!--配置只扫描controller-->
<context:include-filter type="annotation" expression="org.springframework.stereotype.Controller"/>
</context:component-scan>
- 扫描注解
<mvc:annotation-driven/>
- 配置静态资源处理方式
设置Web服务器默认的Servlet处理静态资源,而不是DispatcherServlet处理
<mvc:default-servlet-handler/>
springmvc.xml源码
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd">
<!--配置扫描controller-->
<context:component-scan base-package="com.godgy">
<context:include-filter type="annotation" expression="org.springframework.stereotype.Controller"/>
</context:component-scan>
<!--扫描注解-->
<mvc:annotation-driven/>
<!--不处理静态资源-->
<mvc:default-servlet-handler/>
</beans>
4、测试请求
- 开启Tomcat服务器
在maven的tomcat插件中,启动服务器
![图片[1]-【SSM整合】如何快速构建一个SSM项目②-梦境学习站](https://img.godgy.xyz/phbed/2022/08/14/62f8d21d2130b.jpg)
- 测试post请求
使用apifox或postman等软件发送请求
![图片[2]-【SSM整合】如何快速构建一个SSM项目②-梦境学习站](https://img.godgy.xyz/phbed/2022/08/14/62f8d21dc99ae.jpg)
SSM整合文章
感谢您的来访,获取更多精彩文章请收藏本站。
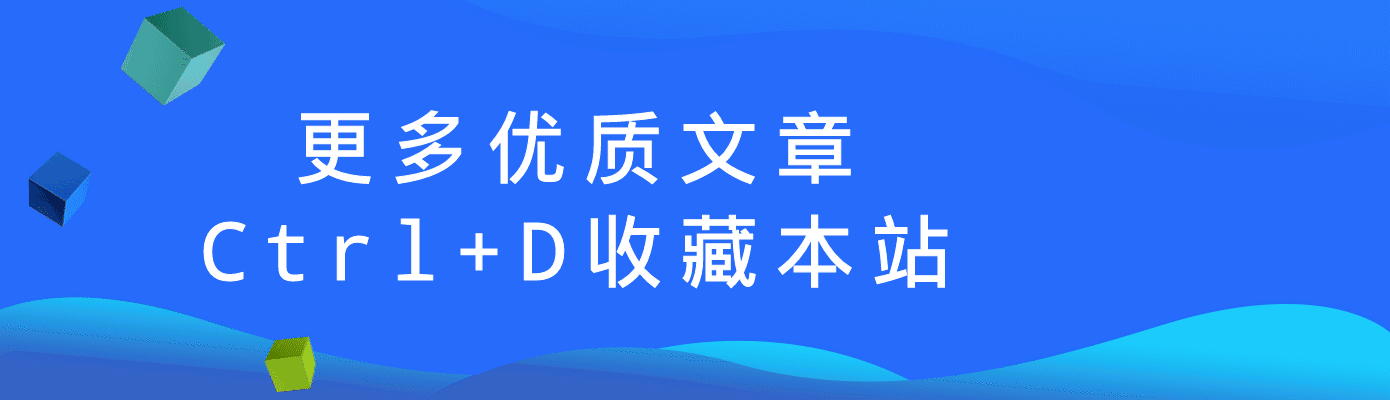
© 版权声明
THE END
暂无评论内容