项目结构
![图片[1]-Spring实战之集成七牛云,实现文件上传下载-梦境学习站](https://blog.godgy.xyz/wp-content/uploads/2022/09/image.png)
代码编写
pom文件
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>com.qiniu</groupId>
<artifactId>qiniu-java-sdk</artifactId>
<version>[7.7.0, 7.7.99]</version>
</dependency>
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>3.14.2</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.5</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>com.qiniu</groupId>
<artifactId>happy-dns-java</artifactId>
<version>0.1.6</version>
<scope>test</scope>
</dependency>
</dependencies>
yml文件
server:
port: 8077
oss:
qiniu:
accessKey:
secretKey:
# 空间名称
bucketName:
# 存储区域
zone: huabei
# 访问域名
domain:
spring:
thymeleaf:
cache: false
配置类
package com.lx.config;
import com.google.gson.Gson;
import com.qiniu.common.Zone;
import com.qiniu.storage.BucketManager;
import com.qiniu.storage.Region;
import com.qiniu.storage.UploadManager;
import com.qiniu.util.Auth;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class QiNiuConfig {
@Value("${oss.qiniu.accessKey}")
private String accessKey;
@Value("${oss.qiniu.secretKey}")
private String secretKey;
@Value("${oss.qiniu.zone}")
private String zone;
/**
* 初始化配置
*/
@Bean
public com.qiniu.storage.Configuration ossConfig(){
switch (zone) {
case "huadong":
return new com.qiniu.storage.Configuration(Region.huadong());
case "huabei":
return new com.qiniu.storage.Configuration(Region.huabei());
case "huanan":
return new com.qiniu.storage.Configuration(Region.huanan());
case "beimei":
return new com.qiniu.storage.Configuration(Region.beimei());
default:
throw new RuntimeException("存储区域配置错误");
}
}
/**
* 认证信息实例
* @return
*/
@Bean
public Auth auth() {
return Auth.create(accessKey, secretKey);
}
/**
* 构建一个七牛上传工具实例
*/
@Bean
public UploadManager uploadManager(com.qiniu.storage.Configuration configuration) {
return new UploadManager(configuration);
}
/**
* 构建七牛空间管理实例
* @param auth 认证信息
* @param configuration com.qiniu.storage.Configuration
* @return
*/
@Bean
public BucketManager bucketManager(Auth auth, com.qiniu.storage.Configuration configuration) {
return new BucketManager(auth, configuration);
}
/**
* Gson
* @return
*/
@Bean
public Gson gson() {
return new Gson();
}
}
工具类
package com.lx.qiniu;
import com.google.gson.Gson;
import com.qiniu.common.QiniuException;
import com.qiniu.http.Response;
import com.qiniu.storage.BucketManager;
import com.qiniu.storage.Configuration;
import com.qiniu.storage.UploadManager;
import com.qiniu.storage.model.DefaultPutRet;
import com.qiniu.util.Auth;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import java.io.IOException;
import java.io.InputStream;
import java.net.URLEncoder;
@Component
public class OssQiNiuHelper {
@Value("${oss.qiniu.bucketName}")
private String bucketName ;
@Value("${oss.qiniu.domain}")
private String fileDomain;
@Autowired
private Configuration configuration;
@Autowired
private UploadManager uploadManager;
@Autowired
private BucketManager bucketManager;
// 密钥配置
@Autowired
private Auth auth;
@Autowired
private Gson gson;
//简单上传模式的凭证
public String getUpToken() {
return auth.uploadToken(bucketName);
}
//覆盖上传模式的凭证
public String getUpToken(String fileKey) {
return auth.uploadToken(bucketName, fileKey);
}
/**
* 上传二进制数据
* @param data
* @param fileKey
* @return
* @throws IOException
*/
public DefaultPutRet upload(byte[] data, String fileKey) throws IOException {
Response res = uploadManager.put(data, fileKey, getUpToken(fileKey));
// 解析上传成功的结果
DefaultPutRet putRet = gson.fromJson(res.bodyString(), DefaultPutRet.class);
System.out.println(putRet.key);
System.out.println(putRet.hash);
return putRet;
}
/**
* 上传输入流
* @param inputStream
* @param fileKey
* @return
* @throws IOException
*/
public DefaultPutRet upload(InputStream inputStream, String fileKey) throws IOException {
Response res = uploadManager .put(inputStream, fileKey, getUpToken(fileKey),null,null);
// 解析上传成功的结果
DefaultPutRet putRet = gson.fromJson(res.bodyString(), DefaultPutRet.class);
System.out.println(putRet.key);
System.out.println(putRet.hash);
return putRet ;
}
/**
* 删除文件
* @param fileKey
* @return
* @throws QiniuException
*/
public boolean delete(String fileKey) throws QiniuException {
Response response = bucketManager.delete(bucketName, fileKey);
return response.statusCode == 200 ? true:false;
}
/**
* 获取公共空间文件
* @param fileKey
* @return
*/
public String getFile(String fileKey) throws Exception{
String encodedFileName = URLEncoder.encode(fileKey, "utf-8").replace("+", "%20");
String url = String.format("%s/%s", fileDomain, encodedFileName);
return url;
}
/**
* 获取私有空间文件
* @param fileKey
* @return
*/
public String getPrivateFile(String fileKey) throws Exception{
String encodedFileName = URLEncoder.encode(fileKey, "utf-8").replace("+", "%20");
String publicUrl = String.format("%s/%s", "http://"+fileDomain, encodedFileName);
long expireInSeconds = 3600;//1小时,可以自定义链接过期时间
String finalUrl = auth.privateDownloadUrl(publicUrl, expireInSeconds);
return finalUrl;
}
}
controller
package com.lx.controller;
import com.lx.qiniu.OssQiNiuHelper;
import com.lx.web.ResultJson;
import com.qiniu.storage.model.DefaultPutRet;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletResponse;
import java.io.FileInputStream;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import java.util.UUID;
@RestController
@RequestMapping("qiniu")
public class OssController {
@Autowired
private OssQiNiuHelper ossQiNiuHelper;
@Value("${oss.qiniu.domain}")
private String fileDomain;
/**
* 七牛云文件上传
*
* @param file 文件
* @return
*/
@PostMapping(value = "/upload")
public ResultJson upload(MultipartFile file) {
if (file == null) {
return ResultJson.fail("上传文件不能为空");
}
try {
FileInputStream fileInputStream = (FileInputStream) file.getInputStream();
String originalFilename = file.getOriginalFilename();
String fileExtend = originalFilename.substring(originalFilename.lastIndexOf("."));
String yyyyMMddHHmmss = new SimpleDateFormat("yyyyMMddHHmmss").format(new Date());
//默认不指定key的情况下,以文件内容的hash值作为文件名
String fileKey = UUID.randomUUID().toString().replace("-", "") + "-" + yyyyMMddHHmmss + fileExtend;
Map<String, Object> map = new HashMap<>();
DefaultPutRet uploadInfo = ossQiNiuHelper.upload(fileInputStream, fileKey);
map.put("fileName", uploadInfo.key);
map.put("originName", originalFilename);
map.put("size", file.getSize());
//七牛云文件私有下载地址(看自己七牛云公开还是私有配置)
map.put("url", "http://"+fileDomain+"/"+ uploadInfo.key);
return ResultJson.ok(map);
} catch (Exception e) {
e.printStackTrace();
return ResultJson.fail(e.getMessage());
}
}
/**
* 七牛云私有文件下载
*
* @param filename 文件名
* @return
*/
@GetMapping(value = "/private/file/{filename}")
public void privateDownload(@PathVariable("filename") String filename, HttpServletResponse response) {
if (filename.isEmpty()) {
return;
}
try {
String privateFile = ossQiNiuHelper.getPrivateFile(filename);
response.sendRedirect(privateFile);
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* 七牛云文件下载
*
* @param filename 文件名
* @return
*/
@RequestMapping(value = "/file/{filename}",method = {RequestMethod.GET})
public void download(@PathVariable("filename") String filename, HttpServletResponse response) {
if (filename.isEmpty()) {
return;
}
try {
String privateFile = ossQiNiuHelper.getFile(filename);
response.sendRedirect("http://"+privateFile);
} catch (Exception e) {
e.printStackTrace();
}
}
}
结果集
package com.lx.web;
import lombok.Data;
@Data
public class ResultJson {
private final static int OK = 200;
private final static int ERR = 500;
private int code;
private String msg;
private Object data;
private ResultJson(int code, String msg, Object data) {
this.code = code;
this.msg = msg;
this.data = data;
}
public static ResultJson ok(String msg, Object data){
return new ResultJson(OK,msg,data);
}
public static ResultJson ok(Object data){
return ok(null,data);
}
public static ResultJson ok(){
return ok(null,null);
}
public static ResultJson fail(String msg,Object data){
return new ResultJson(ERR,msg,data);
}
public static ResultJson fail(Object data){
return ok(null,data);
}
public static ResultJson fail(){
return ok(null,null);
}
}
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>文件上传下载测试</title>
<script type="text/javascript" src="js/jquery1.9.1.min.js"></script>
</head>
<body>
<h1>七牛Oss</h1>
<h3>文件上传测试</h3>
<form action="/qiniu/upload" method="post" enctype="multipart/form-data" target="_blank">
<input type="file" name="file">
<input type="submit" value="上传"/>
<br/>
</form>
<h3>文件下载测试</h3>
<input type="text" id="imgText" style="width: 500px;"/>
<input type="button" placeholder="请输入filekey" onclick="qiNiuImg()" value="测试"/>
<br/>
<img id="img"/>
<hr/>
<script>
function qiNiuImg(){
$('#img').attr('src',"qiniu/private/file/"+$('#imgText').val());
}
</script>
</body>
</html>
测试结果
上传
![图片[2]-Spring实战之集成七牛云,实现文件上传下载-梦境学习站](https://blog.godgy.xyz/wp-content/uploads/2022/09/image-1-1024x292.png)
下载
![图片[3]-Spring实战之集成七牛云,实现文件上传下载-梦境学习站](https://blog.godgy.xyz/wp-content/uploads/2022/09/image-2.png)
感谢您的来访,获取更多精彩文章请收藏本站。
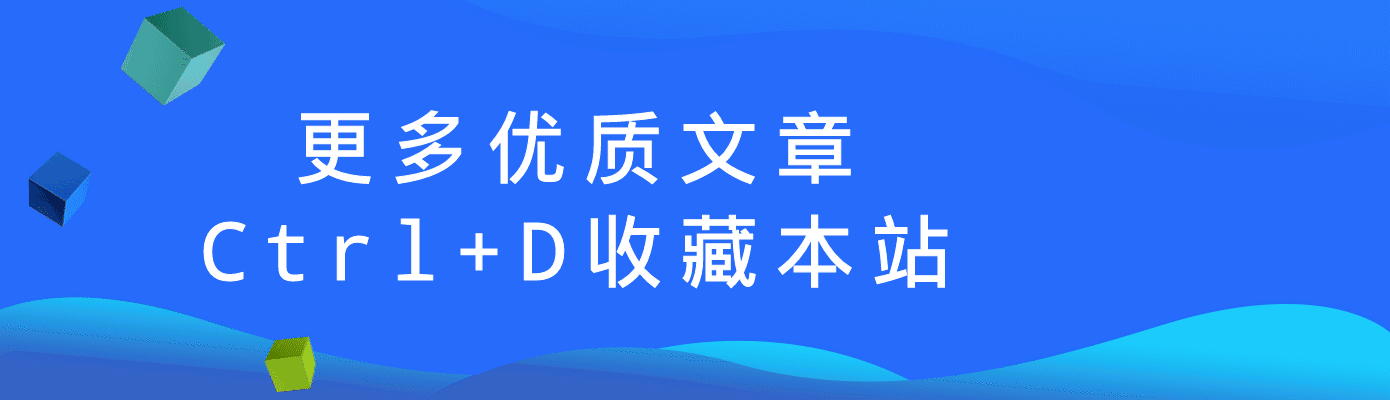
© 版权声明
THE END
暂无评论内容